Lesson 8: Package your JavaScript Code with Functions
You can already add quite a bit of JavaScript functionality to web pages, and that's great. However, what if you decide to implement the same kind of functionality on more than one page, or in different places on the same page?This is where JavaScript functions come into play.
In this lesson, you will learn:
- what functions are and how they are used in JavaScript;
- how to retrieve information from functions;
- how to give information to functions;
- what variable scope is all about.
- get today's date dynamically;
- change the value of an HTML button element dynamically.
Functions: what they are and what they are for
In previous lessons you used JavaScript functions to perform some actions quickly and easily like generating random numbers, writing something on a web page, etc. In fact, JavaScript has a great many built-in functions like write(), alert(), getElementById(), random(), floor(), and several others. Wherever you spot a pair of round brackets there's likely a function in action.JavaScript also gives you the option to craft your own functions.
A function is a way of packaging your JavaScript commands so you can easily reuse them every time you need the same piece of functionality implemented in your website.
This suggests that there are 2 distinct phases to a function:
-
The phase where the function is declared (created);
-
The phase where the function is called (used).
Declare a function
The basic structure of a JavaScript function looks like this://keyword function followed by your //chosen name for the function //Don't forget the round brackets! function functionName() //the code block is inside curly braces { //code you want to run goes here }
Call a function
Once you put a block of code into a function, you can use it anywhere you need that functionality in your website. What you need to do is just call the function.You do this by typing the function name followed by brackets and the function will do its job - just like you did with alert(). Here's how it's done:
//Type the function name (without the keyword function) //Don't forget the round brackets! functionName();
Let's see how this works in practice. Fire off your text editor: it's time to get coding!
Try out: declare your function
Prepare a simple HTML page like the one below. The program we're going to build has the following goals:- to get today's date dynamically when the user clicks a button;
- to display today's date on the web page;
- to change the value attribute of the button after it's clicked: to have the button still displaying Get Date after the date has been displayed looks a bit confusing to the user.
<!DOCTYPE html> <html> <head> <title>Lesson 8: Declare a function</title> </head> <body> <h1>Lesson 8: Declare a function</h1> <div> <h2>Today's date is:</h2> <span id="calendar"></span> <input type="button" id="myButton" value="Get Date" /> </div> <script type="text/javascript"> //Declare your function here function showDate() { //the block of code starts here: //First get all your vars ready //This is how JavaScript retrieves today's date var today = new Date(); //get hold of the calendar span element //where today's date will be inserted var myCalendar = document.getElementById("calendar"); //get hold of the button:you need this when it comes //to change its value attribute var myButton = document.getElementById("myButton"); //insert the date in the span element. //toDateString() changes the date just retrieved //into a user-friendly format for display myCalendar.innerHTML = today.toDateString(); //change the value attribute of the button //to say something more appropriate once the date is displayed myButton.value = "Well done!"; } </script> </body> </html>
Try out: call your function
If you review your program's goals as set out at the beginning, you'll see that all the action takes place after the user clicks the button on the page. This tells us that we need to handle the button's onclick event.If you go back to lesson 3 for a moment, you remember that one way in which this can easily be done is to put some JavaScript code as the value of the HTML element onclick attribute.
Just add an onclick attribute to the button element and plug your showDate() function right in there, like so:
<input type="button" id="myButton" value="Get Date" onclick="showDate();" />
Questions, questions, questions
I know, there's some new stuff in the code samples above. You might be wondering: 1) what's this new Date() business? And 2) What does toDateString() do to the date? Let's tackle each question in turn.-
What's new Date() all about?
The new keyword creates a new instance of a JavaScript object, in this case a Date object based on the user's computer clock. Objects are the topic of the next lesson, and the Date object is the topic of lesson 11. Therefore, I keep things really short at this point.
The important thing you need to know now is that once you've created an instance of the Date object, you've got all sorts of useful functions (called the object's methods) at your fingertips to manipulate date and time.
-
Why did I use toDateString() with the date?
Following on from the previous point, toDateString() is only one of the numerous methods you can use with the Date object. The JavaScript interpreter reads it as follows:
"Hey browser, take the date you've been attached to and make it a bit more human-friendly!"
This is what I mean. Type the following inside enclosing <script> tags of an HTML page: var myDate = new Date(); document.write(myDate);
Save the page and run it in the browser: your date should be displayed in a format like the one below (obviously I expect the date to be different):
Sun Nov 06 2011 14:45:30 GMT+0000 (GMT Standard Time)
Ugly! Luckily, the Date object has its own beauty remedy. Rewrite the previous code snippet as follows: var myDate = new Date(); document.write(myDate.toDateString());
And here's what the date above looks like after its beauty treatment with toDateString():
Sun Nov 06 2011.
Much better, less scary, and far more readable for us humans.
Feed information to and retrieve information from a function
Functions manipulate data: the alert() function has messages to display as its data, the write() function has text to write on the web page as its data, etc.You can also feed data to a function for manipulation. The way you do this is through arguments (or parameters). An argument is placed inside the function's brackets when the function is declared. You can place one or more arguments separated by commas( , ) inside a function.
Here's what the basic structure looks like:
function functionName(arg1, arg2, arg3) { //body of the function goes here }
Finally, one useful thing functions can do with data, once it's been manipulated, is to return it.
For example, take the floor(number) function that you already know from the previous lesson. This function takes in a number argument and returns an integer number.
If your script does something with the returned value then it needs to assign the function to a variable when calling the function.
Let's put this knowledge into practice right away. Create a function and then call it to use its return value in your document.
Declare a function with a parameter
Prepare a simple HTML page and type the following JavaScript code within <script> ... </script> tags:<!DOCTYPE html> <html> <head> <title>Lesson 8: Function Arguments and Return Values</title> </head> <body> <h1>Lesson 8: Function Arguments and Return Values</h1> <script type="text/javascript"> //declare a function with two arguments of type number function addNumbers(num1, num2) { //this is a simple function: // just return the sum of the two number arguments return num1 + num2; //your function ends here } </script> </body> </html>
Call your function and use its return value
Continue on from the previous code. Outside your function, just after the } closing curly brace, type the following://Call your function: create a var //and assign the value returned by your function to it //Also, give a value to the number arguments //by typing 2 comma-separated numbers within brackets var total = addNumbers(3, 5); //Display the returned data on the page document.write(total);
Function arguments are not limited to a specific data type. You can also use strings and booleans, and even other functions. However, we don't want to be too involved at this stage.
Variables inside and outside functions: scope
A variable can have local or global scope on the basis of whether it's declared inside or outside a function block.. But, what does this mean exactly?Simply put, a variable having local scope means that it's visible, or accessible, only within the function in which it lives. No other portion of your code can see a local variable.
On the other hand, a variable having global scope means that it's accessible, that is, it can be used, anywhere in your script.. Let's see this in practice. Get your text editor ready and type the following snippet between opening and closing <script> tags in an HTML page:
//create 2 global variables var message = "outside global message"; var otherGlobalVariable = "other global variable"; //create a function with a local variable //having the same name as the first global variable function getMessage() { var message = "inside local variable"; //the function alerts the message variable alert(message); //and it also alerts the second global variable alert(otherGlobalVariable); //the function ends here } //call the function getMessage(); //alert the message variable //(which one will it be, local or global?) alert(message);
This might seem a bit confusing at first, but it's all a matter of practicing your coding skills as often as you can. The important thing to remember is that, if at times the variables seem not to have the values you expect, it might be a scope-related bug.
Summary
That's all for this lesson. You can now package your JavaScript code into reusable and manageable functions, input and output data via a function, use the Date object to retrieve and display the current date, change the value of an HTML button element on-the-fly, and distinguish between local and global variables.
This is a wide and complex subject and we're only going to scratch the surface in this tutorial series. However, because as soon as you start coding in JavaScript you can't avoid dealing with objects, it's important that you get familiar with some core concepts of OOP.
This short lesson is introductory to the more detailed and practical lessons on specific JavaScript objects that follow.
Here is what you will learn:
What most computer programs do is manipulate, manage, and reuse data of various kinds. Therefore, using the real world object metaphor, contemporary programming languages like JavaScript deal with their virtual environment by populating it with packaged data modelled after the concept of an object.
Everything in JavaScript is an object and you've already used plenty of objects without realizing it. In fact, you're already familiar with:
The answer is: not much differently from the way we humans manipulate real world objects. We do this by interacting with the qualities and capabilities that belong to individual objects.
Let's take a ball as our example. We interact with the ball by means of some of its qualities (its roundness, its hardness, its size, etc.). Also, we interact with the ball on the bais of what we expect the ball's behavior to be like (we expect that we can launch the ball without breaking it, that we can throw it against a wall and it bounces back, etc.).
Similarly, a JavaScript script interacts with its object-modelled data by means of the objects' qualities and behavior. These are called properties and methods.
Properties are values associated with an object. For example, an HTML element object has a value property (like the button object you're familiar with), and an innerHTML property, that you used to add new mark-up to the web page.
Methods represent what an object can do, its behavior, and are very much like functions.
You use the object.property and object.method syntax to interact with JavaScript objects.
Starting from this lesson and for the next 3 lessons, we will be getting up close and personal with some of the most common properties and methods of widely used JavaScript objects: String, Date, Math, and Array. However, because JavaScript as a programming language is mostly made of objects, you will keep learning to use more objects and related properties and methods throughout the rest of this tutorial.
In this lesson you will learn how to use the following properties and methods of the string object:
The beauty of Object Oriented Programming is that we can use all that JavaScript goodness to achieve our script's goals without needing to have any clue whatsoever of the inner workings of those properties and methods. All we need to know is what a property or method can do for us, not how it does it.
For example, if we need to know the length of a piece of text, just using pieceOfText.length will achieve this. This is accomplished without us knowing anything of the programming virtuosity responsible for the power of the length property of the string object.
Let's have a taste of such power.
Here's a basic code snippet to demonstrate its use:
Try out for yourself: insert the code above between enclosing <script> tags and have fun experimenting with it.
Here's a basic code snippet to demonstrate its use:
Have a try: insert the code above between enclosing <script> tags and switch uppercase into lowercase.
Here's a basic code snippet to demonstrate its use:
Experiment on your own: insert the code above between enclosing <script> tags and try matching different string values.
Here's a basic code snippet to demonstrate its use:
Have a go: insert the code above between enclosing <script> tags and try replacing different string values.
Here's a basic code snippet to demonstrate its use:
Have a go on your own: insert the code above between enclosing <script> tags and print the index position of different letters inside the string.
Your JavaScript code will be placed in its own external file and referenced from the HTML document. Separation of concerns, that is, separation between structure (HTML mark-up), appearance (CSS), and behavior (JavaScript), reflects contemporary best practices in web design.
Let's start with the HTML document. This is a simple web page with a textbox, a button, and a div where the JavaScript result will be displayed:
Pay attention to the enclosing <head> tags. These contain a reference to an external JavaScript file named lesson10.js located in the same directory as the HTML file (you're free to place the JavaScript file in its own directory, if you prefer. However, make sure this is reflected in the src value of your <script> tag in the HTML document).
Also, take note of the fact that the elements that are going to play a role in our script all have an id attribute. This gives JavaScript a hook on those elements and the data they will eventually contain.
Now open the JavaScript file lesson10.js and type the following code:
Save all your files and run the HTML page in the browser. You should see something similar to the example page indicated in the link above.
If you click the button without entering any value in the textbox, an alert will pop up inviting you to enter an answer.
If you get right the first half ("Harry") - indexOf() returns 0 ("Harry" is at position 0, which is the start of the index) - but not the latter half ("Potter") - indexOf() returns -1 (it finds no corresponding value inside the string), you get an alert letting you know that your answer is almost right.
If you enter the correct answer, well you'll get a prize.
Finally, if your answer is totally wrong, your application will say so and give you the correct answer.
The above code sample employs all the JavaScript tools you learned in this lesson and most of those you encountered in previous lessons. Experiment with it as much as you can. If you have doubts, leave a message in our JavaScript forum.
The really new stuff in the guessing game application is the way JavaScript functions work from an external file. This is the technique I'm going to use for our Try out exercises in most of the remaining part of this tutorial - at least until jQuery makes its appearance. This means that you'll be getting plenty of practice with it.
Here we continue to explore the power of JavaScript objects: we will be looking into the magical virtues of the Date object.
Here's what you will do in this lesson:
The Date object exposes plenty of methods to manipulate dates and times, some of which we're going to examine here.
I recommend you try out all the demos below by inserting the code snippets between enclosing <script> tags in an HTML page (for such short demos we can get away with not using an external JavaScript file ). Also, feel free to experiment with each demo as much as possible before moving on to the next example.
After a Date object is created, you can use its methods to get and set dates and times.
You already had occasion to taste the power of the Math object back in lesson 7, where you used its random() method to generate a random number between 0 and 1, and its floor() method to round down the resulting random number.
The Math object allows you to write scripts that perform complex mathematical tasks in the blink of an eye.
In this lesson you will use:
Let's quickly refresh our school math. Here are some definitions:
PI is the ratio of the circumference of a circle to the diameter.
If you need this value in your JavaScript program, use Math.PI. Just try this one out between enclosing <script> tags of an HTML page:
Keeping in mind that the radius is half the diameter, and that the formula to calculate the circumference is PI * diameter, our JavaScript statement to calculate the circumference given the radius will be: 2 * (Math.PI * radius).
First things first: prepare an HTML document like this one:
The HTML page above references an external JavaScript file, lesson12_tryout1.js, and contains an inputbox, a button, and a paragraph where the result of the calculation will be displayed. Make sure these 3 crucial elements have an id value so your JavaScript script will have an easy to reach hook on those elements.
Now create a JavaScript document and name it lesson12_tryout1.js. This file will contain 3 functions:
Save all your files and run the HTML page in the browser. You should see something like the page indicated by following the example link above.
If you click the button without entering any value in the inputbox, or if you enter a letter rather than a number, the program asks you to enter a number value.
As you click the button, the calculation is performed and the result is displayed on the page.
If a negative number is entered as argument, for instance Math.sqrt(-5), the function returns NaN, that is, a value that JavaScript does not treat as a number.
Brushing up on our school math, the square root of 25, for example, is that number that multiplied by itself yields 25 as a result, that is, 5.
The formula is: square of n = n * n. JavaScript performs this calculation automatically. Here's a quick demo: type the following code between enclosing <script> tags of an HTML page:
Now create the lesson12_tryout2.js file. This file will contain 3 functions:
Save all files and preview your work in a browser. You should see something similar to the example indicated by following the link above.
Enter a number into the inputbox and click the button. If you enter anything but a number into the inputbox (or if you leave the inputbox empty), you'll be alerted by a popup box asking you to enter a number. If all goes well, the square root of the number you enter in the inputbox will be displayed on the page.
If the application is not working, check your typing, especially letter casing, brackets, and semi-colons ( ; ). If you have any doubts, just drop us a line in the forum dedicated to this tutorial.
Lesson 9: A Gentle Introduction to Objects
Object Oriented Programming (OOP) is a programming model used by most contemporary programming languages. JavaScript offers significant object oriented capabilities, has its own built-in objects, and offers you the option of creating your own custom objects.This is a wide and complex subject and we're only going to scratch the surface in this tutorial series. However, because as soon as you start coding in JavaScript you can't avoid dealing with objects, it's important that you get familiar with some core concepts of OOP.
This short lesson is introductory to the more detailed and practical lessons on specific JavaScript objects that follow.
Here is what you will learn:
- the concept of object in web programming;
- what object properties are;
- what object methods are.
What is an object in Geeky talk?
Real world objects are things like books, cars, balls, etc. We humans deal with the world around us by interacting with and manipulating things, that is, objects.What most computer programs do is manipulate, manage, and reuse data of various kinds. Therefore, using the real world object metaphor, contemporary programming languages like JavaScript deal with their virtual environment by populating it with packaged data modelled after the concept of an object.
Everything in JavaScript is an object and you've already used plenty of objects without realizing it. In fact, you're already familiar with:
- the Document object that you used to print your JavaScript messages on the web page;
- the Math object, that you used to generate random numbers and round off decimals;
- HTML elements like the button object that you used to manipulate its value attribute;
- the Date object that you used to retrieve the current date.
Properties and methods
But how exactly does JavaScript manipulate objects?The answer is: not much differently from the way we humans manipulate real world objects. We do this by interacting with the qualities and capabilities that belong to individual objects.
Let's take a ball as our example. We interact with the ball by means of some of its qualities (its roundness, its hardness, its size, etc.). Also, we interact with the ball on the bais of what we expect the ball's behavior to be like (we expect that we can launch the ball without breaking it, that we can throw it against a wall and it bounces back, etc.).
Similarly, a JavaScript script interacts with its object-modelled data by means of the objects' qualities and behavior. These are called properties and methods.
Properties are values associated with an object. For example, an HTML element object has a value property (like the button object you're familiar with), and an innerHTML property, that you used to add new mark-up to the web page.
Methods represent what an object can do, its behavior, and are very much like functions.
How do you associate an object with a property or a method?
If you wondered what that odd-looking ( . ) dot notation in document.write() or Math.random(), and so on, meant in previous lessons, here's the answer.You use the object.property and object.method syntax to interact with JavaScript objects.
//use the random() method of the Math object Math.random(); //use the write() method of the document object document.write(); //use the length property of the string object myStringText.length;
Summary
This lesson has been a short introduction to the concept of JavaScript objects, their properties and methods.Lesson 10: JavaScript Objects - Strings
In the previous lesson you were introduced to the concept of Object Oriented Programming (OOP). You're now familiar with the use of objects and their properties and methods in JavaScript programming.Starting from this lesson and for the next 3 lessons, we will be getting up close and personal with some of the most common properties and methods of widely used JavaScript objects: String, Date, Math, and Array. However, because JavaScript as a programming language is mostly made of objects, you will keep learning to use more objects and related properties and methods throughout the rest of this tutorial.
In this lesson you will learn how to use the following properties and methods of the string object:
- length property;
- toLowerCase()/toUpperCase();
- match();
- replace();
- indexOf().
The String object
You've had plenty of practice with the string object through this tutorial. In fact, the JavaScript interpreter reads any piece of text enclosed in quotes ' ' (or double quotes " ") as an instance of the string object. This puts the magic of all the properties and methods belonging to the string object in our hands.The beauty of Object Oriented Programming is that we can use all that JavaScript goodness to achieve our script's goals without needing to have any clue whatsoever of the inner workings of those properties and methods. All we need to know is what a property or method can do for us, not how it does it.
For example, if we need to know the length of a piece of text, just using pieceOfText.length will achieve this. This is accomplished without us knowing anything of the programming virtuosity responsible for the power of the length property of the string object.
Let's have a taste of such power.
How to use length
The length property of the string object contains the number of characters (including spaces) in the text value of a string.Here's a basic code snippet to demonstrate its use:
//create and initialize a string variable var myString = "Hello JavaScript" //apply the length property to the string document.write(myString.length); //JavaScript will print 16: //spaces are included in the count //If the string is empty length returns 0
How to use toUpperCase()
The toUpperCase() method of the string object turns the text value of a string into, well ... uppercase letters. Its companion toLowerCase() does the opposite: it turns the text value of a string into lowercase letters.Here's a basic code snippet to demonstrate its use:
//create and initialize a string variable var myString = "Hello JavaScript" //apply the toUpperCase() method to the string document.write(myString.toUpperCase()); //JavaScript will print HELLO JAVASCRIPT //Try using myString.toLowerCase() on your own
How to use match()
The match() method of the string object is used to search for a specific value inside a string.Here's a basic code snippet to demonstrate its use:
//create and initialize a string variable var myString = "Hello JavaScript" //apply the match() method to the string. //match(stringToMatch) takes the value to search for as argument document.write(myString.match("JavaScript")); //JavaScript will print JavaScript //If no match is found the method returns null
How to use replace()
The replace() method of the string object is used to replace a value for another value inside a string.Here's a basic code snippet to demonstrate its use:
//create and initialize a string variable var myString = "Hello JavaScript" //apply the replace() method to the string. //replace() takes 2 arguments and returns the new string value: //replace(valueToReplace, newValue) document.write(myString.replace("JavaScript", "World")); //JavaScript will print Hello World
How to use indexOf()
The indexOf() method of the string object is used to know the position of the first found occurrence of a value inside a string.Here's a basic code snippet to demonstrate its use:
//create and initialize a string variable var myString = "Hello JavaScript" //apply the indexOf() method to the string. //indexOf() takes in the value to look for in the string as argument //and returns the position number (index) //indexOf(valueToFind) document.write(myString.indexOf("Java")); //JavaScript will print 6 //indexOf() includes spaces and starts counting at 0 //if no value is found the method returns -1
Try out: guessing game
You're going to build a simple guessing game application using all the new knowledge you've acquired in this lesson. Your application is expected to check a name entered by the user and respond accordingly:Your JavaScript code will be placed in its own external file and referenced from the HTML document. Separation of concerns, that is, separation between structure (HTML mark-up), appearance (CSS), and behavior (JavaScript), reflects contemporary best practices in web design.
Let's start with the HTML document. This is a simple web page with a textbox, a button, and a div where the JavaScript result will be displayed:
<!DOCTYPE html> <html> <head> <title>Lesson 10: JavaScript Objects - Strings</title> <script type="text/javascript" src="lesson10.js"></script> </head> <body> <h1>Lesson 10: JavaScript Objects - Strings</h1> <h2>What's the name of the wizard boy in J.K. Rowling's novels?</h2> <p>Your answer: <input type="text" id="txtName" /></p> <p><input type="button" value="Submit your answer" id="btnAnswer" /> <p id="message"></p> </body> </html>
Also, take note of the fact that the elements that are going to play a role in our script all have an id attribute. This gives JavaScript a hook on those elements and the data they will eventually contain.
Now open the JavaScript file lesson10.js and type the following code:
//This is the function that initializes our program function init() { //Store a reference to the HTML button element in a variable: //use the id of the HTML button element to do this var myButton = document.getElementById("btnAnswer"); //use the onclick event of the button //and assign the value of the getAnswer() function to it. //This function performs the main job in your application //and runs after the user clicks the button on the page. //Take note: getAnswer is assigned without brackets. //This is so because otherwise getAnswer() would be called //as soon as the page loads (and we don't want that). myButton.onclick = getAnswer; //the init function ends here } //Assign the init() function to the onload event: //this event fires when the HTML page is loaded in the browser. //Take note: init is assigned without brackets onload = init; //Now write the getAnswer() function function getAnswer() { //Create all the vars you need to manipulate your data: //secretName stores the correct answer the user is expected to guess: var secretName = "Harry Potter"; //Turn the value of secretName into lower case: //you do this because you're going to compare this value //to the value entered by the user of your application. //Given that users might type the answer either in upper or lower case, //reducing the relevant text values to the same casing automatically //ensures that only the content and not also the letter case plays a role in the comparison. var secretNameLower = secretName.toLowerCase(); //Get the value the user types into the textbox var myTextBox = document.getElementById("txtName"); var name = myTextBox.value; //Also turn the value entered by the user to lower case var nameLower = name.toLowerCase(); //Get a reference to the HTML paragraph that will display your result //after the script has run by storing its id value in a var var message = document.getElementById("message"); //These are the test cases your application needs to evaluate //and respond to: if the user clicks the button but did not //enter any value in the textbox: if(nameLower.length <= 0) { alert("I didn't quite catch your answer. Please enter an answer"); } //If the user gets right the first half but not the latter half of the name: //Take note of the use of the logical operator && //(go back to lesson 5 if you need to revise logical operators) else if(nameLower.indexOf("harry") == 0 && nameLower.indexOf("potter") == -1) { alert("Almost there: think again"); } //If the secret name and the name entered by the user match: else if(nameLower.match(secretNameLower)) { alert("You got it!"); message.innerHTML = "Congratulations, you win!"; } //Default case - if the user types in the wrong answer: else { alert("Wrong!"); message.innerHTML = "Sorry. The correct answer is: "; message.innerHTML += name.replace(name, "Harry Potter"); } //the getAnswer() function ends here }
If you click the button without entering any value in the textbox, an alert will pop up inviting you to enter an answer.
If you get right the first half ("Harry") - indexOf() returns 0 ("Harry" is at position 0, which is the start of the index) - but not the latter half ("Potter") - indexOf() returns -1 (it finds no corresponding value inside the string), you get an alert letting you know that your answer is almost right.
If you enter the correct answer, well you'll get a prize.
Finally, if your answer is totally wrong, your application will say so and give you the correct answer.
The above code sample employs all the JavaScript tools you learned in this lesson and most of those you encountered in previous lessons. Experiment with it as much as you can. If you have doubts, leave a message in our JavaScript forum.
The really new stuff in the guessing game application is the way JavaScript functions work from an external file. This is the technique I'm going to use for our Try out exercises in most of the remaining part of this tutorial - at least until jQuery makes its appearance. This means that you'll be getting plenty of practice with it.
Lesson 11: JavaScript Objects - Date Object
In the previous lesson, you had a go at manipulating text by taking advantage of the powerful properties and methods of the String object.Here we continue to explore the power of JavaScript objects: we will be looking into the magical virtues of the Date object.
Here's what you will do in this lesson:
- create a Date object;
- set dates;
- compare dates.
The Date object
In lesson 8 you learned how to retrieve the current date and display it on the web page in user-friendly format. This could be done dynamically thanks to the JavaScript goodness offered by the Date object.The Date object exposes plenty of methods to manipulate dates and times, some of which we're going to examine here.
I recommend you try out all the demos below by inserting the code snippets between enclosing <script> tags in an HTML page (for such short demos we can get away with not using an external JavaScript file ). Also, feel free to experiment with each demo as much as possible before moving on to the next example.
Create a Date object
You create a date object by using one of the following ways://First way: //the var today is initialized with a Date object //containing the current date and time //on the basis of the user's computer //use toLocaleDateString() to adjust the date //to the local time zone and format it automatically var today = new Date().toLocaleDateString(); //Second way: //you pass a millisecond argument that starts at 1970/01/01: //new Date(milliseconds) var date = new Date(1000).toLocaleDateString(); //On my system this outputs: 01 January 1970 //Third way: //You pass a string as argument: //new Date(dateString) var date = new Date("10 November, 2011").toLocaleDateString(); //On my system this outputs: 10 November 2011 Fourth way: //new Date(year, month, day, hours, minutes, seconds, milliseconds) //only year and month are required, //the others are optional arguments and //where not present 0 is passed by default //Below you pass the year in a 4-digit format, and the month in number form: //months are represented by numbers from 0 (January) to 11 (December) var date = new Date(2011, 10).toLocaleDateString(); //On my system this outputs: 01 November 2011
Use getDate() to retrieve a date
//Create a Date object containing the current date and time var myDate = new Date(); //use getDate() to extract the day of the month document.write(myDate.getDate()); //At the time of writing, this prints 11 //days of the month range from 1 to 31
Use getTime() to retrieve a time
//Create a Date object containing the current date and time var myTime = new Date(); //use getTime() to extract the time document.write(myTime.getTime()); //At the time of writing, this prints 1321021815555 //This is the millisecond representation of the current //date object: the number of milliseconds between //1/1/1970 (GMT) and the current Date object
Get Date object components
Once you have a Date object, one interesting thing you can do with it is to get its various components. JavaScript offers some interesting methods to do just that.Use getFullYear() to extract the year component
//Create a Date object containing the current date and time var myDate = new Date(); //extract the year component and print it on the page document.write(myDate.getFullYear()); //At the time of writing, this prints 2011
Use getMonth() to extract the month component
//Create a Date object containing the current date and time var myDate = new Date(); //extract the month component and print it on the page document.write(myDate.getMonth()); //At the time of writing, this prints 10 //which represents the month of November - months are represented //with numbers starting at 0 (January) and ending with 11 (December)
Use getDay() to extract the day component
//Create a Date object containing the current date and time var myDate = new Date(); //extract the day component and print it on the page document.write(myDate.getDay()); //At the time of writing, this prints 5 //which represents Friday - days are represented //with numbers starting at 0 (Sunday) and ending with 6 (Saturday)
Use getHours() and getMinutes() to extract time components
//Create a Date object containing the current date and time var myDate = new Date(); //extract the hours component var hours = myDate.getHours(); //extract the minutes component var minutes = myDate.getMinutes(); //format and display the result on the page: //check the minutes value is greater than 1 digit //by being less than 10 if (minutes < 10) { //If it's just a one digit number, then add a 0 in front minutes = "0" + minutes; } var timeString = hours + " : " + minutes; document.write(timeString); //Based on my computer clock, this prints: //14 : 44
Set dates
You can also set dates and times as easily as calling the appropriate methods. Here are a few examples.Use setFullYear() to set a specific date
In this demo, you will set a specific date and then retrieve its day component for display//Create a Date object containing the current date and time var myDate = new Date(); //set the Date object to a specific date: 31 October, 2011 var mySetDate = myDate.setFullYear(2011, 9, 31); //Now retrieve the newly set date var mySetDay = myDate.getDay(mySetDate); //mySetDay will contain a number between 0 - 6 (Sunday - Saturday). //Use mySetDay as test case for a switch statement //to assign the corresponding week day to the number value switch (mySetDay) { case 0: mySetDay = "Sunday"; break; case 1: mySetDay = "Monday"; break; case 2: mySetDay = "Tuesday"; break; case 3: mySetDay = "Wednesday"; break; case 4: mySetDay = "Thursday"; break; case 5: mySetDay = "Friday"; break; case 6: mySetDay = "Saturday"; break; } //display the result on the page document.write("The 31st October 2011 is a " + mySetDay); //If you check the date on a calendar, you'll find that //the 31st Oct 2011 is indeed a Monday
Compare 2 dates
In this demo you will compare 2 Date objects.//Create a Date object var myDate = new Date(); //set the Date object to a specific date: 31 October, 2011 var mySetDate = myDate.setFullYear(2011, 9, 31); //Create a second Date object var now = new Date(); //Make the comparison: if the set date is bigger than today's date if (mySetDate > now); { //the set date is in the future document.write("The 31st October is in the future"); } //if the set date is smaller than today's date, it's in the past else { document.write("The 31st October is in the past"); }
Summary
In this lesson you looked into some of the methods of the Date object and had the chance to experiment with them.Lesson 12: JavaScript Objects - Math Object
We continue on our journey through JavaScript built-in objects by offering a quick overview of the Math object.You already had occasion to taste the power of the Math object back in lesson 7, where you used its random() method to generate a random number between 0 and 1, and its floor() method to round down the resulting random number.
The Math object allows you to write scripts that perform complex mathematical tasks in the blink of an eye.
In this lesson you will use:
- Math.PI to calculate the circumference of a circle;
- Math.sqrt() to calculate a square root value.
- parseInt()/parseFloat() to convert strings to numbers;
- isNaN() to check whether a value is or is not a number;
Math.PI
This property stores the value of PI, a mathematical constant that amounts to approximately 3.14159.Let's quickly refresh our school math. Here are some definitions:
Circle
A circle is a shape with all points the same distance from the center: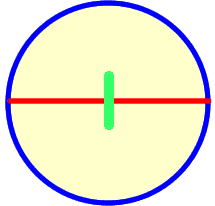
- the round border surrounding the shape (the color blue in the graphic above), is the circle circumference;
- the line cutting the circle horizontally in half (the color red in the graphic above) is the circle diameter;
- each half of the diameter represents a radius.
If you need this value in your JavaScript program, use Math.PI. Just try this one out between enclosing <script> tags of an HTML page:
//store and display the value of PI: var pi = Math.PI; document.write(pi);
Try out: use Math.PI to find out the circumference of a circle
It's time for this lesson's first try out exercise. We're going to build a simple application where the user enters a number representing the radius of a circle, clicks a button, and the program calculates the circumference and displays it on the page.Keeping in mind that the radius is half the diameter, and that the formula to calculate the circumference is PI * diameter, our JavaScript statement to calculate the circumference given the radius will be: 2 * (Math.PI * radius).
First things first: prepare an HTML document like this one:
<!DOCTYPE html> <html> <head> <title>Lesson 12: JavaScript Objects - Math Object</title> <script type="text/javascript" src="lesson12_tryout1.js"></script> </head> <body> <h1>Lesson 12: Circumference calculator</h1> <p>Enter length of radius: <input type="text" id="txtRadius" /></p> <p><input type="button" id="btnSubmit" value="Calculate circumference" /></p> <p id="result"></p> </body> </html>
Now create a JavaScript document and name it lesson12_tryout1.js. This file will contain 3 functions:
- init() initializes the script by binding the processAnswer() function to its appropriate event, that is, the btnSubmit onclick event;
- processAnswer() checks the answer submitted by the user, calls the function that performs the calculation, and displays the result;
- getCircumference(rad) gets passed the radius value as argument, performs the actual calculation, and returns the circumference.
//init() function binds the processAnswer() function //to the onclick event of the button function init() { var myButton = document.getElementById("btnSubmit"); myButton.onclick = processAnswer; } /*********************************************/ //Bind the init function to the onload event onload = init; /*********************************************/ //This function checks the user's input, //calls the function that performs the calculation, //and displays the result function processAnswer() { //store a reference to the inputbox var inputBox = document.getElementById("txtRadius"); //get the radius value entered in the inputbox //make sure to convert the string (text) to a number: //calculations can only be performed with numbers, //and the value in the inputbox is processed as string. //parseInt(string) and parseFloat(string) //take a string as input and return an integer //or a floating point number respectively. var radius = parseInt(inputBox.value); //get a reference to the paragraph to display result var result = document.getElementById("result"); //create and initialize a var to store the result var resultString = ""; //Perform some validation on the user's input: //if the value entered by the user is not a number //alert the user. isNaN(value) takes //a value as input and returns true if the value is not a number, //it returns false if the value is a number. //the expression below is short for: //if (isNaN(radius) == true) if (isNaN(radius)) { alert("Please, enter a number"); } else { //if the user has correctly entered a number, //call the function that performs the calculation //and display the result on the page var circ = getCircumference(radius); resultString = "Circumference is: " + circ; result.innerHTML = resultString; } } /*********************************************/ //This is the function that performs the calculation: //it takes the radius as input and returns the circumference function getCircumference(rad) { var circumference = 2 * (Math.PI * rad); return circumference; }
If you click the button without entering any value in the inputbox, or if you enter a letter rather than a number, the program asks you to enter a number value.
As you click the button, the calculation is performed and the result is displayed on the page.
Math.sqrt()
Math.sqrt(number) takes a number as argument and returns the square root of that number.If a negative number is entered as argument, for instance Math.sqrt(-5), the function returns NaN, that is, a value that JavaScript does not treat as a number.
Brushing up on our school math, the square root of 25, for example, is that number that multiplied by itself yields 25 as a result, that is, 5.
The formula is: square of n = n * n. JavaScript performs this calculation automatically. Here's a quick demo: type the following code between enclosing <script> tags of an HTML page:
var squareRoot = Math.sqrt(25); document.write("The square root of 25 is: " + squareRoot); //This should print: //The square root of 25 is: 5
Try out: square root calculator
You will build a simple square root calculator. Here are the requirements for this little application:- the user will be able to enter a number value into an inputbox;
- the user will be able to click a button that calculates the square root of the number entered into the inputbox;
- the result will be displayed on the web page.
<!DOCTYPE html> <html> <head> <title>Lesson 12: JavaScript Objects - Math Object</title> <script type="text/javascript" src="lesson12_tryout2.js"></script> </head> <body> <h1>Lesson 12: Square root calculator</h1> <p>Enter a number: <input type="text" id="txtNumber" /></p> <p><input type="button" id="btnSubmit" value="Calculate square root" /></p> <p id="result"></p> </body> </html>
- init() initializes the script by binding the displaySquare() function to its appropriate event, that is, the btnSubmit onclick event;
- displaySquare() checks the answer submitted by the user, calls the function that performs the calculation, and displays the result;
- calculateSquare(input) gets passed the number value entered by the user as argument, performs the actual calculation, and returns the the square root.
//write the function to initialize the script function init() { //Bind function that processes the result to //the onclick event of the button var myButton = document.getElementById("btnSubmit"); myButton.onclick = displaySquare; } /**************************************************/ //Bind the init() function to the onload event onload = init; /**************************************************/ //Here we call the function that performs //the square root calculation, then we format and //display the result function displaySquare() { //we store the value from the inputbox into a var. //Notice how we concatenate several methods //in one statement (be careful with those brackets). //If you find it a bit confusing, store a reference to the inputbox //in its own var and then use it to extract its value property //and finally pass it as argument of parseInt(), like so: //var inputBox = document.getElementById("txtNumber"); //var inputVal = parseInt(inputBox.value); var inputVal = parseInt(document.getElementById("txtNumber").value); //we store a reference to the paragraph //where the result will be displayed var result = document.getElementById("result"); //we create the var that stores the result message var resultMessage = ""; //we ensure the input value is a number: //if the user did not enter a number, we send an alert if (isNaN(inputVal)) { alert("Please, enter a number"); } else { //If the input is in correct format, we call //the function that performs the calculation var squareVal = calculateSquare(inputVal); //this is a nested if statement: it's inside another //if statement to perform further checks: //if squareVal stores a value (if the calculation was successful) - //- this is short for: if (squareVal == true) if (squareVal) { //we build this message to the user: resultMessage = "Square root of " + inputVal + " is " + squareVal; } //else, that is, if the calculation was not successful else { //we build a different message to the user: resultMessage = "Sorry, an error occurred"; } } //finally, we display the message using innerHTML result.innerHTML = resultMessage; } /**************************************************/ //This function calculates the square root: //it takes in the number entered by the user //and returns the result of the calculation function calculateSquare(input) { var squareVal = Math.sqrt(input); return squareVal; }
Enter a number into the inputbox and click the button. If you enter anything but a number into the inputbox (or if you leave the inputbox empty), you'll be alerted by a popup box asking you to enter a number. If all goes well, the square root of the number you enter in the inputbox will be displayed on the page.
If the application is not working, check your typing, especially letter casing, brackets, and semi-colons ( ; ). If you have any doubts, just drop us a line in the forum dedicated to this tutorial.