JavaScript gives you the freedom to add interactivity and responsiveness to your web pages.
The aim of this tutorial is to provide you with a thorough, yet accessible introduction to JavaScript using snappy explanations and practical tasks to try out right from the start.No prior knowledge of JavaScript is assumed, but because JavaScript sits within and manipulates web pages, in order to be able to follow along, you should already be familiar with HTML and CSS. If you are new to either or both, you’re advised to step through our HTML and CSS tutorials first.
JavaScript is a lightweight, easy to learn, scripting language. It’s used on almost every website to respond to user actions, validate web forms, detect browser support, and much more.
JavaScript is a web programming language, that is, a language that enables you, the designer of your website, to control how a web page behaves. This makes JavaScript crucially different from HTML, the language that gives structure to your web documents, and CSS, the language that controls the appearance of web pages.
If you know other programming languages such as PHP, most programming concepts and basic JavaScript syntax will sound quite familiar to you. However, if this is not the case, don’t worry: by following along and experimenting with the code, at the end of this hands-on tutorial you’ll be able to spruce up your static web pages with fun effects and fantastic responsiveness for the joy of your website visitors.
If you need help along the way, don’t forget to turn to forums. This is where you meet the real experts who are willing and ready to offer tips, suggestions and advice.What is needed?
To bring to life your web pages with JavaScript all you need is a text editor and an updated, standard-compliant browser of your choice, such as Internet Explorer 9, Firefox, Chrome, Safari, or Opera, to mention just the most popular ones.Basic free text editors that ship with your operating system such as Notepad (on Windows) and Text Edit (on Mac) will do just fine. However, text editors that offer programming languages support, such as the free Notepad++ (for Windows users) and TextWrangler (for Mac users), might be a great resource when ploughing through more than a few lines of code.
Lesson 1: What is JavaScript?
The first thing that creates some confusion about JavaScript is its name. In fact, one of the most common questions students raise when approaching JavaScript is:"Is JavaScript the same as Java?".Clearing up this basic but important question is our first order of business in this lesson, but by no means the only one. By the end of this lesson you will also know:
- the difference between JavaScript and Java;
- what JavaScript can and cannot do.
Are JavaScript and Java the same thing?
No, they are not.Java (developed by Sun Microsystems) is a powerful and much more complex programming language in the same category as C and C++.
JavaScript was created by Brendan Eich at Netscape and was first introduced in December 1995 under the name of LiveScript. However, it was rather quickly renamed JavaScript, although JavaScript’s official name is ECMAScript, which is developed and maintained by theECMA (European Computer Manufacturer's Association) International organization.
JavaScript is a scripting language, that is, a lightweight programming language that is interpreted by the browser engine when the web page is loaded.
The fact that the JavaScript interpreter is the browser engine itself accounts for some inconsistencies in the way your JavaScript-powered page might behave in different browsers. But don't worry: thankfully, well-established techniques and powerful JavaScript libraries such as jQuery (which will be introduced in later lessons) are here to make things wonderfully easier on us.
Things you can't do with JavaScript
You can't force JavaScript on a browser.
Conclusion: unlike what happens with languages that run on the server, such as PHP, you never fully know for sure the impact that the browser your website visitors are going to use will have on your script, or whether your visitors will choose to turn JavaScript support off.
You can't access or affect resources from another internet domain with JavaScript.

This is exactly the kind of nasty situation that the Same Origin Policy is designed to prevent. Conclusion: your JavaScript script can only access resources in your website.
You can't access server resources with JavaScript.
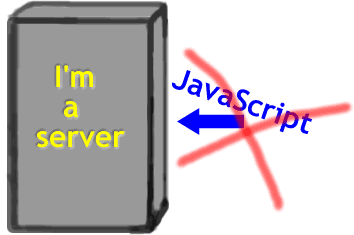
Zillion things you can do with JavaScript
With JavaScript you can:

Say you want to display a nice thank you message to a user who has just submitted a comment form on your website. Obviously, the message needs to be added after the user has submitted the form.
You could let the server do that. However, if your website is very busy and your server processes hundreds of forms a day, it might take a little while for your thank you message to appear to the user.
Here's JavaScript to the rescue. Because JavaScript runs in the user's browser, the thank you note can be added and displayed on the page almost instantaneously, making your website users happy.

Web environments are dynamic, things happen all the time: the web page loads in the browser, the user clicks a button or moves the mouse over a link, etc. These are called events (which will be the topic of lesson 3).
With JavaScript you can make the page immediately react to these events the way you choose: for example, by showing or hiding specific page elements, by changing the background color, etc.

You can use a JavaScript script to detect the visitor’s browser, or, even better, you can detect what features a certain browser does or does not support. Depending on the browser and its capabilities, you can choose to load a page specifically tailored to that kind of browser (lesson 14).

A JavaScript script is great if you want to create cookies so that your visitors can enjoy a personalized experience the next time they visit your website (lesson 15).

You can use a JavaScript script to validate form data before the form is submitted to a server. This saves the server from extra processing (lesson 16).
And much ... much more.
Learning JavaScript will enable you to add cool animation effects to your web pages without using an external Flash plug-in, use the newest features of HTML5 such as canvas (to draw directly on your web page) and drag and drop capabilities, integrate your website with external web services such as Facebook, Twitter, etc.
Summary
In this lesson you learned what JavaScript is, who invented it, and that the body responsible for its maintenance and continuous development is ECMA. Now you know what you cannot do with JavaScript, but also the great things that you can do with it.Lesson 2: Your First JavaScript
In this lesson you are going to learn:- how to embed your JavaScript in the HTML page;
- how to reference your JavaScript from a separate file;
- how to comment your JavaScript code and why it is recommended;
- how to create your first JavaScript-powered web page.
The HTML
To insert a JavaScript script in an HTML page, you use the <script> ... </script> tag. Don't forget the closing </script> tag! Now get ready to fire off your text editor of choice and let's get coding!Let's start with a basic HTML page, like this one:
<!DOCTYPE html> <html> <head> <title>My first JavaScript page</title> </head> <body> </body> </html>
Embed JavaScript in the HTML page
The <script> tag and its type attribute tell the browser: "Hey, browser! There's a script coming up, and it's a JavaScript script."You can do this either in the <head> section, as follows:
<!DOCTYPE html> <html> <head> <title>My first JavaScript page</title> <script type="text/javascript"> //JavaScript code goes here </script> </head> <body> </body> </html>
<!DOCTYPE html> <html> <head> <title>My first JavaScript page</title> </head> <body> <script type="text/javascript"> //JavaScript code goes here </script> </body> </html>
It mostly comes down to personal preference. However, because most of the times you'll want your JavaScript code to run after the web page and all its resources, e.g., stylesheets, graphics, videos, etc., have finished loading in the browser, I suggest you dump your JavaScript <script> tag at the bottom of your page.
Comments, comments, comments
One final thing to note about both code snippets above is the two forward slashes // before the text "JavaScript code goes here". This is how you comment a one-line JavaScript code.When a comment spans over more than one line, you use /* Comment goes here */ to delimit a comment, just like you do in a stylesheet. Here's how it's done:
<!DOCTYPE html> <html> <head> <title>My first JavaScript page</title> </head> <body> <script type="text/javascript"> /* JavaScript code goes here */ </script> </body> </html>
Insert JavaScript in a separate file
If your script is longer than a few lines, or you need to apply the same code to several pages in your website, then packaging your JavaScript code into a separate file is your best bet.In fact, just like having your CSS all in one place, well away from HTML code, having your JavaScript in its own file will give you the advantage of easily maintaining and reusing your scripts.
Here's how it's done:
<!DOCTYPE html> <html> <head> <title>My first JavaScript page</title> <script type="text/javascript" src="yourjavascript.js"></script> </head> <body> </body> </html>
Your first JavaScript-powered page: Hello World
Without further ado, let's see if JavaScript works in your browser.Try out: embedded JavaScript in an HTML page
Between the <script> and </script> tags either in the <head> or the <body> of your HTML document, add the following line of code, just after the comment:<!DOCTYPE html> <html> <head> <title>My first JavaScript page</title> </head> <body> <script type="text/javascript"> //JavaScript code goes here alert('Hello World!'); </script> </body> </html>
Just notice the semicolon ( ; ) at the end of the statement. This is important: it tells the JavaScript interpreter that the statement is finished and whatever comes next is a different statement.
Now save your work and run the page in your favorite browser. You'll see an alert box popping up on page load. This is how the page looks in Firefox:
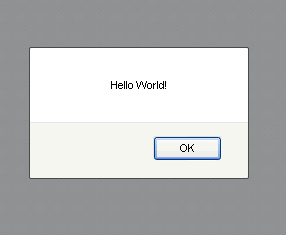
Make sure your <script>...</script> tags are there, that the text between the brackets is surrounded by quotes (' '), and that there is a semicolon ( ; ) at the end of the statement. Then try again.
Try out: JavaScript in a separate file
Create a new document in your text editor and save it as "helloworld.js". Important: the file extension has to be .js (this is the appropriate JavaScript file extension).In the new document, paste in the JavaScript command from the previous example (no need to type the <script> ... </script> tags here):
alert('Hello World!');
<!DOCTYPE html> <html> <head> <title>My first JavaScript page</title> <script type="text/javascript" src="helloworld.js"></script> </head> <body> </body> </html>
If the code doesn't work, in your HTML document check that the filepath to the JavaScript file is correct, the filename spelling is accurate, and double-check that you've added a closing </script> tag. In helloworld.js make sure your JavaScript command is typed exactly the same as in the sample code above, then try again.
Summary
You've actually learned a lot in this lesson. You know how and where to include JavaScript in your web page, how to comment your JavaScript code and why this is a good idea, and finally you've just seen your web page come alive with your first JavaScript script.Admittedly, an alert box saying "Hello World!" looks a bit dumb, but even alerts can be useful to quickly and easily test that JavaScript is enabled in your browser and your code is working.
Lesson 4: Variables and Constants
Variables and constants are like containers where you store data and values for processing in JavaScript.The difference between a variable and a constant is this: once you give a value to a constant the value is meant to be kept unchanged throughout the script. In all circumstances where you reasonably foresee that the original value is modified through the script, by all means use a variable as your storage room. In fact, you're going to use variables most of the times.
In this lesson, you're going to learn:
- how to create variables and constants;
- how to assign values to variables and constants;
- how to use variables in your code;
- how to name variables and constants correctly.
How to create variables and constants
You declare, that is, create variables and constants in a similar way.Here's how you declare a variable:
/*To declare a variable you give it a name preceded by the JavaScript keyword var*/ var amountDue;
The variable declaration ends with a ( ; ) semicolon, which makes it a self-contained statement.
Also, because JavaScript is case sensitive, every time you refer to the amountDue variable, make sure you keep the letter case exactly the same as the original declaration (this particular notation is called camelCase because it looks like a camel's hunch).
Here's how you declare a constant:
/*To declare a constant you give it a name preceded by the JavaScript keyword const Also take note: const is not supported by Internet Explorer use var instead: it's safer*/ const taxRate;
How to assign values to variables
After you create a variable, you must assign (give) a value to it (or said in Geeeky talk "initialize a variable") . The ( = ) equal sign is called assignment operator: you assign the value of what is on the right side of the = sign to whatever is on the left side of the = sign. Notice: you cannot perform operations with empty variables.Ready to fire off your text editor? Let's get coding!
Prepare a basic HTML document with the JavaScript code illustrated below:
<!DOCTYPE html> <html> <head> <title>Lesson 4: Variables and Constants</title> </head> <body> <h1>Lesson 4: Variables and Constants</h1> <script type="text/javascript"> //Create a variable var amountDue; /* Assign a value to it: you do not know the value yet so for now it is 0 */ amountDue = 0; /* Create 2 more vars and assign a value to each at the same time */ var productPrice = 5; var quantity = 2; /* Assign a value to amountDue by multiplying productPrice by quantity */ amountDue = productPrice * quantity; /* Notice how the value of amountDue has changed from 0 to 10 - -Alert the result to check it is OK Notice: you do not use ' ' with var name in alert() */ alert(amountDue); </script> </body> </html>
That's great! Try experimenting with the code above. For example, change the value of quantity and productPrice, or just come up with your own variables.
How to name variables (and constants)
Choose descriptive names for your variables (var amountDue; rather than var x;): this way your code will be more readable and understandable.While this can be called good programming practice (but also common sense), there are also syntax rules (yes, just like any natural language) when it comes to naming variables, and they must be obeyed, at least if you want your JavaScript script to work.
Keep an eye on the following simple rules when you name variables:
1) The first character must be a letter, an ( _ ) underscore, or a ( $ ) dollar sign:

5total is wrong: var name cannot start with a number
2) Each character after the first character can be a letter, an ( _ ) underscore, a ( $ ) dollar sign, or a number:

3) Spaces and special characters other than ( _ ) and $ are not allowed anywhere:
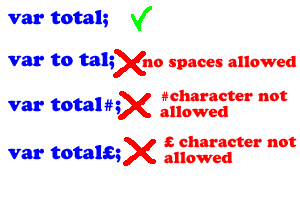
- to tal is wrong: spaces are not allowed;
- total# is wrong: # character is not allowed;
- total£ is wrong: £ character is not allowed.
Summary
Variables and constants are the building blocks of any programming language. In this lesson you learned the part they play in JavaScript, how you declare and assign values to them, how to name your variables correctly, and you also had a taste of using variables in JavaScript.In the next lesson you're going to learn about JavaScript operators and do more and more practice with variables.
Lesson 3: Events
The web is a dynamic environment where a lot of things happen. Most appropriately, they're called events. Some of these events, like a user clicking a button or moving the mouse over a link, are of great interest to a JavaScript coder.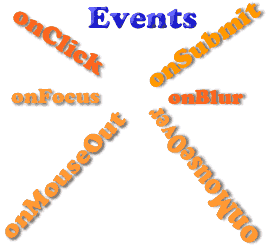
- what events are;
- what JavaScript can do with events and how.
What are events?
Events are occurrences taking place in the context of the interactions between web server, web browser, and web user.For instance, if you're on a web page and click on a link, there are at least 3 important events being triggered:
- onClick event: triggered by you as you click the link;
- onUnload event: triggered by the web browser as it leaves the current web page;
- onLoad event: triggered by the browser as the new web page content is loaded.
Which events should I focus on from a JavaScript point of view?
It all depends on the JavaScript program you're writing, that is, on the objectives you want to achieve with your script.However, the most common events you're likely to deal with in your JavaScript are:
- onLoad/onUnload;
- onClick;
- onSubmit (triggered by the user submitting a form);
- onFocus / onBlur (triggered by a user as, for example, she clicks in a textbox or clicks away from a textbox respectively);
- onMouseOver / onMouseOut (triggered by the user moving the mouse over or away from an HTML element respectively).
However, for the purposes of this tutorial, you're mostly going to come across the core events listed above.
What do I do with events from a JavaScript point of view?
As you write a JavaScript program, events become interesting because they give your script a hook for gaining control on what happens in the web page.Once your script gets hold of the hook provided by the event, your script is boss. The jargon for this is event-driven programming: an event happens and JavaScript handles it, for instance by displaying an alert box with a message for the user.

Hey, an onClick event going on!
How does JavaScript handle events?
In this tutorial you've already hooked an event handler to an event. More precisely, you attached the alert() command to the onLoad event.The alert() command is a command which is part of the JavaScript language. The JavaScript interpreter in the browser translates it along these lines:
"Hey, browser, display an alert box that contains the message typed within the () enclosing brackets"(Notice: JavaScript is case sensitive. If you write Alert instead of alert, your script won't work!).
As you saw in the previous lesson, simply by writing a JavaScript statement between <script> ... </script> tags achieves the execution of that statement as the onLoad event fires up.
However, your JavaScript scripts are capable of doing more interesting stuff when they handle events in response to user actions, for example an onClick event. How do we do that?
Try out: handle an onClick event with JavaScript
The browser already has its own ways of handling events. For instance, when a page has loaded, the browser fires the onLoad event and displays the page contents; when a user clicks a link, the browser communicates to the server to access the requested page, etc. These are called default actions.The fun of being in charge, though, is not to let the browser do what it likes, but of letting JavaScript do its job and decide what's to be done.
The simplest way to attach an event handler to an event is to insert the required JavaScript code within the HTML element that produces the event. Let's have a go by simply preventing the browser default action as the user clicks a link on the page. Fire off your text editor and let's get coding!
Prepare a new basic HTML document displaying a simple link like the one shown below:
<!DOCTYPE html> <html> <head> <title>Lesson 3: Events and Event Handlers</title> </head> <body> <h1>Lesson 3: Events and Event Handlers</h1> <a href="http://html.net">Click Me!</a> </body> </html>
Now go back to your HTML document and type the following JavaScript command within the <a> tag, as follows:
<!DOCTYPE html>
<html>
<head>
<title>Lesson 3: Events and Event Handlers</title>
</head>
<body>
<h1>Lesson 3: Events and Event Handlers</h1>
<a href="http://html.net" onclick="alert('Going anywhere? Not so fast!'); return false;">Click Me!</a>
</body>
</html>
What's just happened there?
That's how easy it was to take control of a link with JavaScript! All you did was to insert a couple of JavaScript statements to the onclick attribute of the HTML <a> tag.You already know about the good old alert() command, so I'll skip over it. The return false; command tells the JavaScript interpreter to return a value, in this case the value equals false, which prevents the browser from performing its default action. You'll be using this little command quite often in your JavaScript programming life.
You handle the other events listed above in the same way: just insert the JavaScript command as the value of the onfocus, onblur, onmouseover, onmouseout and onsubmit attributes of an HTML element.
Summary
That'll be all for this lesson. You learned what events are, why events are important in JavaScript programming, and how to use event handlers to let JavaScript be in control of your web page behavior.But this is just a tiny taste of what JavaScript can do once it's in charge.
Lesson 5: Smarter Scripts with Operators
In the previous lesson you already employed an assignment operator ( = ) and an arithmetic operator, specifically the multiplication operator ( * ), to write a basic JavaScript shopping cart script.
We can easily see that to do something useful with JavaScript, we need a way to manipulate data and variables. We do this with operators.
In this lesson you are going to learn how to use:
- arithmetic operators;
- the + sign to concatenate text (concatenation operator);
- comparison operators;
- logical operators.
Arithmetic operators
As you might have guessed, arithmetic operators are used to perform arithmetic operations between values or variables. Here's a table for your reference.If x = 20, y = 5, and z = result, we have:
Operator | Java Script Example | Result |
---|---|---|
Addition: + | z = x + y | z = 25 |
Subtraction: - | z = x - y | z = 15 |
Multiplication: * | z = x * y | z = 100 |
Division: / | z = x / y | z = 4 |
Modulus: % | z = x / y | z = 0 |
Increment: ++ | z = ++x | z = 21 |
Decrement -- | z = --x | z = 19 |
Modulus: the remainder left over after division.
Increment: take a number and add 1 to it.
Decrement: take a number and subtract 1 from it.
Time to get coding! Get your hands on the text editor and prepare a new HTML document like the one below:
Try out: add 2 values and print the result
<!DOCTYPE html> <html> <head> <title>Lesson 5: Operators and Comparisons</title> </head> <body> <h1>Lesson 5: Operators and Comparisons</h1> <script type="text/javascript"> //Create and initialize your variables var result = 0; var firstNum = 20; var secondNum = 5; //Addition: result = 25 result = firstNum + secondNum; //write result on the page document.write(result); </script> </body> </html>
"Hey browser, get the value within brackets and print it on the HTML document!"In our case the value is a variable, therefore no ( ' ' ) quotes are used to enclose it. If you want to print some text instead, the command must be: document.write('some text.');. It's all very similar to the alert() command you've been using so far.
Now experiment with the code sample above by trying out all the other arithmetic operators and printing the result on the page.
Concatenation operator
If you want to add pieces of text together to form one long line of text, use the + sign. In Geeky talk a piece of text is called string, and it appears enclosed either in (' ') quotes or (" ") double-quotes (remember the 'Hello World' text you used in the alert() command? That is an instance of string).Try out: concatenate strings and print a message on the page
<!DOCTYPE html> <html> <head> <title>Lesson 5: Operators and Comparisons</title> </head> <body> <h1>Lesson 5: Operators and Comparisons</h1> <script type="text/javascript"> //Create and initialize your variables var firstText = "Hello"; var secondText = "World!"; //Resulting value of assignment is Hello World! var message = firstText + ' ' + secondText; //write result on the page document.write(message); </script> </body> </html>
Now get some practice concatenating strings before moving on.
Comparison operators
Often you need to compare different values and make your JavaScript program take different directions accordingly.For example, you're coding a JavaScript script for a shopping cart application. At one point, your script will have a statement saying something along these lines: if the total amount to be paid is greater than or equal to $50 apply a 5% discount, if it's less than or equal to $50 do not apply 5% discount. Don't be impatient, you will learn how to code this kind of conditions in the next lesson.
It's here that comparison operators, such as equal to, less than, etc. enter the scene. Here below are listed all comparison operators for your reference.
If x = 10 we have:
Operator | What is it? | Example |
---|---|---|
== | equal to | x == 5 is false |
=== | exactly equal to value and type | x === 10 is true x === "10" is false |
!= | not equal | x != 2 is true |
> | greater than | x > 20 is false |
< | less than | x < 20 is true |
>= | greater than or equal to | x >= 20 is false |
<= | less than or equal to | x <= 20 is true |
Logical operators
You use logical operators when you need to determine the logic between certain values.Going back to the shopping cart script example, you might want your script to apply a 5% discount if the following 2 conditions are both true: a given product costs more than $20 and is purchased before the 31st of December.
Here come logical operators to the rescue. Given that x = 10 and y = 5:
Operator | What is it? | Example |
---|---|---|
&& | and | (x < 20 && y > 1) is true both conditions must be satisfied |
|| | or | (x == 5 || y == 5) is true at least 1 condition must be satisfied |
! | not | !(x == y) is true |
Questions, questions, questions
The tables above are self-explanatory, except for the following 2 questions:- When you talk about === , what do you mean by equality of value and type?
- What's the difference between ( = ), ( == ), and ( === ) ?
Answer to question 1.
Values are the specific data, either directly in your JavaScript statements or contained in JavaScript variables. For example: var price = 5;
What's the type?
The type, or more precisely the data type, is the way JavaScript classifies data. You've come across 2 data types, that is, number and string (text). A third data type is Boolean, that is, true and false statements.
Therefore, when you compare 2 values using ( === ), the 2 values are compared on the basis of both their value and their data type:
var firstNum = 4; var secondNum = 4; //this is true: both values are 4 //and both values are of type number firstNum === secondNum; //let's use a string data type. A string uses ' '. var stringNum = '4'; //Now === is false: 4 and '4' are different types firstNum === stringNum;
Answer to question 2.
The ( = ) operator is used to assign or give a value to a variable. It is not a sign for equality.The ( == ) and ( === ) operators instead, do stand for equality. They do not assign values to variables. ( == ) compares only values, ( === ) compares both values and data type.
Summary
You've made it all the way through this lesson, congratulations! Now your scripts are not limited to just popping up messages. They start to be smart: they can make calculations, comparisons, and set truth conditions to evaluate their data.Lesson 6: Even Smarter Scripts with if…else and switch
Now that our JavaScript code can calculate, compare, and determine true and false conditions, things start looking a lot more interesting.The days of dumb message boxes popping up are long gone, the new era of smart message boxes has begun.
In this lesson you're going to inject the power of choice into your scripts with conditional statements. These statements enable your script to evaluate given conditions on the basis of which to execute one or more actions. More specifically, you're going to learn how to use:
- if statements;
- if ... else statements;
- if ... else if ... else statements;
- switch statements.
if Statement
The if keyword is used in JavaScript to perform a basic test for the truth or falsity of a given condition and execute a piece of code accordingly. Here's its structure://if the condition in brackets is true if (condition) { //do some stuff here }
Let's translate the code
Translated into English, the if statement above says:"Hey browser, if the condition in the round brackets is true, execute the commands between those odd curly braces. If the condition is not true, then ignore everything and move on!"Important: do not forget either the brackets ( ) or the braces { }.
if ... else statement
Use this statement to execute a command if the condition is true and another command if the condition is false. Here's its structure://if the condition is true if (condition) { //do this stuff } //otherwise else { //do this other stuff }
if ... else if ... else statement
Use this statement if you want your code to evaluate several conditions on the basis of which to execute the appropriate commands. Here's its structure://if the condition is true if (condition) { // do this stuff } //if this other condition is true instead else if (condition) { // do this other stuff } //if none of the above condition is true else { // do this instead }
switch statements
In alternative to an if ... else if ... else statement you can switch to a ... well a switch statement. Here's its basic structure://condition to evaluate switch(condition) { //in this case do this case 1: execute code block 1 //stop here: no need to keep going break; //in this other case do that case 2: execute code block 2 //stop here: no need to keep going break; //otherwise fall back on this default: code to execute when all previous conditions evaluate to false }
Try out: check product price with if
<!DOCTYPE html> <html> <head> <title>Lesson 6: Even Smarter Scripts with if…else and switch</title> </head> <body> <h1>Lesson 6: if statements</h1> <script type="text/javascript"> //store 5% discount value in a var var discountRate = 0.05; //store price of 1 apple in a var with a value of 2 var applePrice = 2; //store quantity value in a var var quantity = 5; //declare a var for the discounted total value //because we don't know the value we initialize it with 0 var discountedTotal = 0; //store the value of the total in a var //you use the ( * ) multiplication operator to calculate the total //and the ( = ) assignment operator to assign the value to the var var total = quantity * applePrice; //use if to check the total is entitled to a discount //if total is greater or = to 10 it's entitled if (total >= 10) { alert("You're entitled to a discount!"); //apply 5% discount on the total //and assign it to discountedTotal var //Take note: multiplication is in brackets because it has to be //performed before subtraction (remember the good old school math?) discountedTotal = total - (discountRate * total); //create a var to store text to display to user var infoText = "You'll get your delicious apples at $" + discountedTotal; //you add more text to the same infoText var with a shortcut assignment operator //this technique is useful when you have long text strings to manipulate infoText += " instead of at $" + total; //display message to the user document.write(infoText); } </script> </body> </html>
Questions, questions, questions
It's almost like I can hear you saying something like:Hey, what's going on there? What's that += sign all about?Here's the answer:
Shortcut operators
When you add several values to a variable, a shortcut operator is very handy.The expression x += 2 is the same as x = x + 2.
As you can see, the given value of x is taken as the starting point, 2 is added to it, and the resulting value is reassigned to x. Using a shortcut operator spares you from having to retype x.
When applied to the code snippet above, the expression infoText += " instead of at $" is the same as infoText = infoText + " instead of at $". If we simply use an ( = ) assignment operator in this case, the text already stored in infoText will be wiped out by the new value. But this is not what we want in this script.
Handy shortcuts are also available to all other mathematical operators:
- -=
- *=
- /=
- %=
Try out: check product price with if ... else
Just after the closing curly brace } from the previous example, add the following snippet.else { alert("Sorry, you're not entitled to a discount"); var infoText = "Your delicious apples cost $" + total; document.write(infoText); }
Try out: check product price with if ... if else ... else
Still using the previous example, between the first if block and the last else block, insert the following else if block.//if the total equals to 8 else if (total == 8) { alert("Just add a couple more apples and get your discount!"); var infoText = "Be a sport, buy a bit more and pay a bit less"; document.write(infoText); }
Your code can detect if the purchase qualifies for a discount, if it almost qualifies for a discount, and finally if the discount is inapplicable by a long shot. In each case, your code can take appropriate action.
Have fun practicing with if ... else if ... else statements, and shortcut operators.
Try out: days of the week with switch
Now get a fresh HTML document ready and type the following code block:<!DOCTYPE html> <html> <head> <title>Lesson 6: Even Smarter Scripts with if…else and switch</title> </head> <body> <h1>Lesson 6: switch</h1> <script type="text/javascript"> //pick a day of the week and store it in a var var today = "Friday"; //check today var value and act accordingly switch(today) { //in case value corresponds to Saturday or to Sunday do this: case "Saturday": case "Sunday": alert("It's the weekend: great!"); document.write("Today is " + today); //no need to keep going: break; //in case value corresponds to Friday do this case "Friday": alert("Almost weekend time!"); document.write("Today is " + today); //no need to keep going break; //in all other cases do this instead default: alert("Just an ordinary weekday: keep up the good work"); document.write("Today is " + today); } </script> </body> </html>
Lesson 7: Leave Boring Repetitive Stuff to JavaScript with loops
Your JavaScript scripts can do all sort of clever stuff like calculating discounts, comparing values, and making choices. However, don't forget we're dealing with machine language, and machines are very good at doing some things us humans would keep a mile off if only we could.
One really boring thing most of us would happily avoid doing is repetitive stuff. You might find it hard to believe this, but JavaScript loves repetition. So much so that it has a special construct for it: the loop.
Here's what you will learn in this lesson:
- the for loop;
- the while loop;
- the do ... while loop.
- use getElementById(element Id) to manipulate an HTML element with JavaScript;
- generate random numbers with random() and floor(number);
- insert HTML mark-up dynamically with innerHTML.
Loops
At times you will have to repeat some piece of code a number of times:for instance: a web page has 10 radio buttons and your script needs to find out which radio button was checked by the user.
To do so, your script has to go over each single radio button and verify whether its checked attribute is set to true or false. Does this mean having to write this kind of verification code 10 times?
Thankfully, it does not. You dump the code in a loop once, and it's going to execute any number of times you set it to.
Any loop is made of 4 basic parts:
-
the start value
An initial value is assigned to a variable, usually called i (but you can call it anything you like). This variable acts as counter for the loop.
-
the end value or test condition
The loop needs a limit to be set: either a definite number (loop 5 times) or a truth condition (loop until this condition evaluates to true). Failing this, you run the risk of triggering an infinite loop. This is very bad: it's a never-ending repetition of the same code that stops users' browsers from responding. Avoid infinite loops at all costs by making sure you set a boundary condition to your loops;
-
the action or code to be executed
You type a block of code once and it'll be executed the number of times between your start value and end value;
-
the increment
This is the part that moves the loop forward: the counter you initialize has to move up (or down in case you opt for looping backwards). As long as the loop does not reach the end value or the test condition is not satisfied, the counter is incremented (or decremented). This is usually done using mathematical operators.
The for Loop
This kind of loop, well ... loops through a block of code a set number of times. Choose a for loop if you know in advance how many times your script should run.Here's its basic structure:
//loop for the number of times between start value //and end value. Increment the value each time //the limit has not been reached. for (var=startvalue; var<=endvalue; var=var+increment) { //code to be executed }
The while Loop
If you don't know the exact number of times your code is supposed to execute, use a while loop.With a while loop your code executes while a given condition is true; as soon as this condition evaluates to false, the while loop stops.
Here's its basic structure:
//loop while initial value is less than or equal to end value //Take note: if the condition evaluates to false from the start, //the code will never be executed while (variable <= endvalue) { //code to be executed }
do ... while Loop
This kind of loop is similar to the while loop. The difference between the two is this:In the case of the while loop, if the test condition is false from the start, the code in the loop will never be executed.
In the case of the do ... while loop, the test condition is evaluated after the loop has performed the first cycle. Therefore, even if the test condition is false, the code in the loop will execute once.
Here's the basic structure of a do ... while loop:
//the command is given before the test condition is checked do { //code to be executed } //condition is evaluated at this point: //if it's false the loop stops here while (variable <= endvalue)
Try out: add random number of images with a for loop
Time to get coding: prepare a fresh HTML document with a div tag and an id of "wrapper" and add the following JavaScript magic:<!DOCTYPE html> <html> <head> <title>Lesson 7: Leave Boring Repetitive Stuff to JavaScript with Loops</title> </head> <body> <h1>Lesson 7: for Loop</h1> <div id="wrapper"></div> <script type="text/javascript"> //Grab the html div by its id attribute //and store it in a var named container var container = document.getElementById("wrapper"); //use Math.random to generate a random number //between 0 and 1. Store random number in a var var randomNumber = Math.random(); //use Math.floor() to round off the random number //to an integer value between 0 and 10 and store it in a var var numImages = Math.floor(randomNumber * 11); //just for testing purposes, lets alert the resulting random number alert(numImages); //the loop will run for the number of times indicated //by the resulting random number for (var i=1; i <= numImages; i++) { //code to be executed: //create a var and store a string made of a new HTML img element //and the iteration variable i (use + concatenation operator): //i contains the iteration number //Take note:make sure the img src corresponds to a real image file var newImage = '<img src="loop.gif" alt="#" />' + i; //use the container var that stores the div element //and use innerHTML to insert the string stored in the newImage var //Use shortcut assignment += so previous values do not get wiped off container.innerHTML += newImage; } </script> </body> </html>

Questions, questions, questions
I know, there's a lot to ask about the code sample above. More specifically: 1) What's this document.getElementById(element Id)? 2) What are Math.random() and Math.floor(number)? And finally, 3) what's innerHTML? Let's tackle each question in turn:-
Get hold of HTML elements with document.getElementById(element Id)
JavaScript can perform all sorts of magic on any HTML element once it gets hold of it. This can be done in more than one way. However, the most straightforward way is by using the document.getElementById(element Id) command. The JavaScript interpreter reads it as follows:
"Hey browser, find the HTML element with the id name like the one in brackets."
Because JavaScript usually does something with the element, this gets conveniently stored inside a variable. What you did in the sample code above was to grab hold of the div with the id attribute named wrapper and store it in a variable named container.
-
Generate random numbers with Math.random() and Math.floor(number)
JavaScript has built-in commands to perform complex mathematical operations quickly and easily.
Math.random() generates a random number between 0 and 1. Try it out on your own by typing var randomNum = Math.random(); alert(randomNum); inside a <script> tag.
Math.floor(number) rounds a number downwards to the nearest integer, and returns the result. The JavaScript interpreter reads it as follows:
"Hey browser, take the number in brackets and round it down to the nearest integer number."
Try it out on your own by typing var roundedNumber = Math.floor(6.2); alert(roundedNumber); inside a <script> tag. Keep changing the number in brackets and see how JavaScript returns the corresponding integer number.
In the previous example, you used Math.floor() together with Math.random(number) to generate a random integer number between 0 and 10 (you typed 11 rather than 10 because the round number returned by Math.random() starts at 0 not 1).
-
Add new mark-up inside HTML elements with innerHTML
A JavaScript script can make HTML elements appear and disappear, change place or color, and much more.
There's more than one way to add new mark-up in your HTML document, but using innerHTML makes it very easy and straightforward. The JavaScript interpreter reads the command as follows:
"Hey browser, take the string to the right of the assignment operator and insert it into the HTML mark-up."
What you did in the sample code above was to take the variable storing the wrapper div element and applying innerHTML to it to insert an img tag inside the div.
Try out: add images to the page with a while loop
Use the same HTML document from the previous example, but delete all the JavaScript between the <script> ... </script> tags. Now, type in the following JavaScript code:<script type="text/javascript"> //Grab the html div by its id attribute //and store it in a var named container var container = document.getElementById("wrapper"); //create the var containing the counter //and give it an initial value of 0 var numImages = 0; //start the loop: while the number of images is less than or //equal to 10 (loop starts at 0 not 1, so type 9 not 10), //keep cycling and increase the counter by 1 while (numImages <= 9) { //this is the block of code to be executed: //build the string to insert in the HTML document //and store it in the newImage var //Take note:make sure the img src corresponds to a real image file var newImage = '<img src="loop.gif" alt="#" />' + numImages; //use the container var that stores the div element //and use innerHTML to insert the string stored in the newImage var //Use shortcut assignment += so previous values do not get wiped off container.innerHTML += newImage; //increase the counter by 1 using the increment operator numImages ++; } </script>

Run a while loop with a false condition
Now make just a small change to the code above: replace var numImages = 0; with var numImages = 12;. Now the initial condition is false from the start: the counter is a number greater than 10.Save your work, run the page and see what happens. If all works fine, nothing at all should happen: the loop doesn't even get started.
Try out: add images to the page with a do ... while loop
Use the same HTML document as the previous example. Also, the JavaScript code is very similar to the previous example: simply move the while (condition) outside the action block and type do in its place, like the example below:<script type="text/javascript"> var container = document.getElementById("wrapper"); var numImages = 0; do { //this block gets executed first: var newImage = '<img src="loop.gif" alt="#" />' + newImage; container.innerHTML += newImage; numImages ++; } //here's the condition to evaluate: //the first cycle has already executed. while (numImages <= 9); </script>

Run a do ... while loop with a false condition
Make just a small change to the code above: once again, replace var numImages = 0; with var numImages = 12;. Now the initial condition is false from the start: the counter is a number greater than 10.Save your work, run the page and see what happens. Unlike what happened with the while loop, now you should see one image displayed on the web page: the loop has completed the first cycle and only afterwards the test condition is evaluated. In this instance the condition is false and the loop stops after the first iteration completes.